Lets import Data in a minute
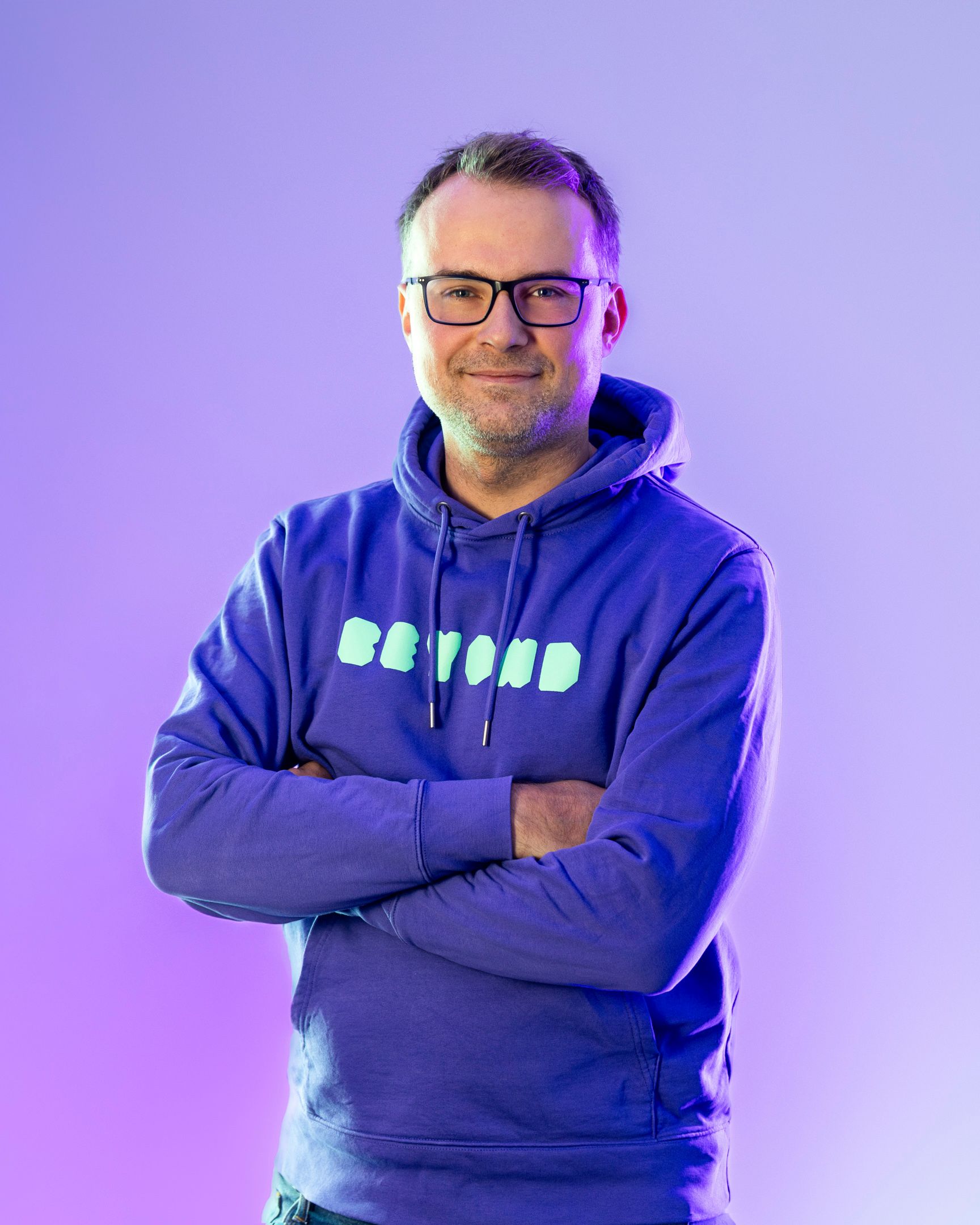
Daniel Gorski
CEO
2 Min. Lesezeit
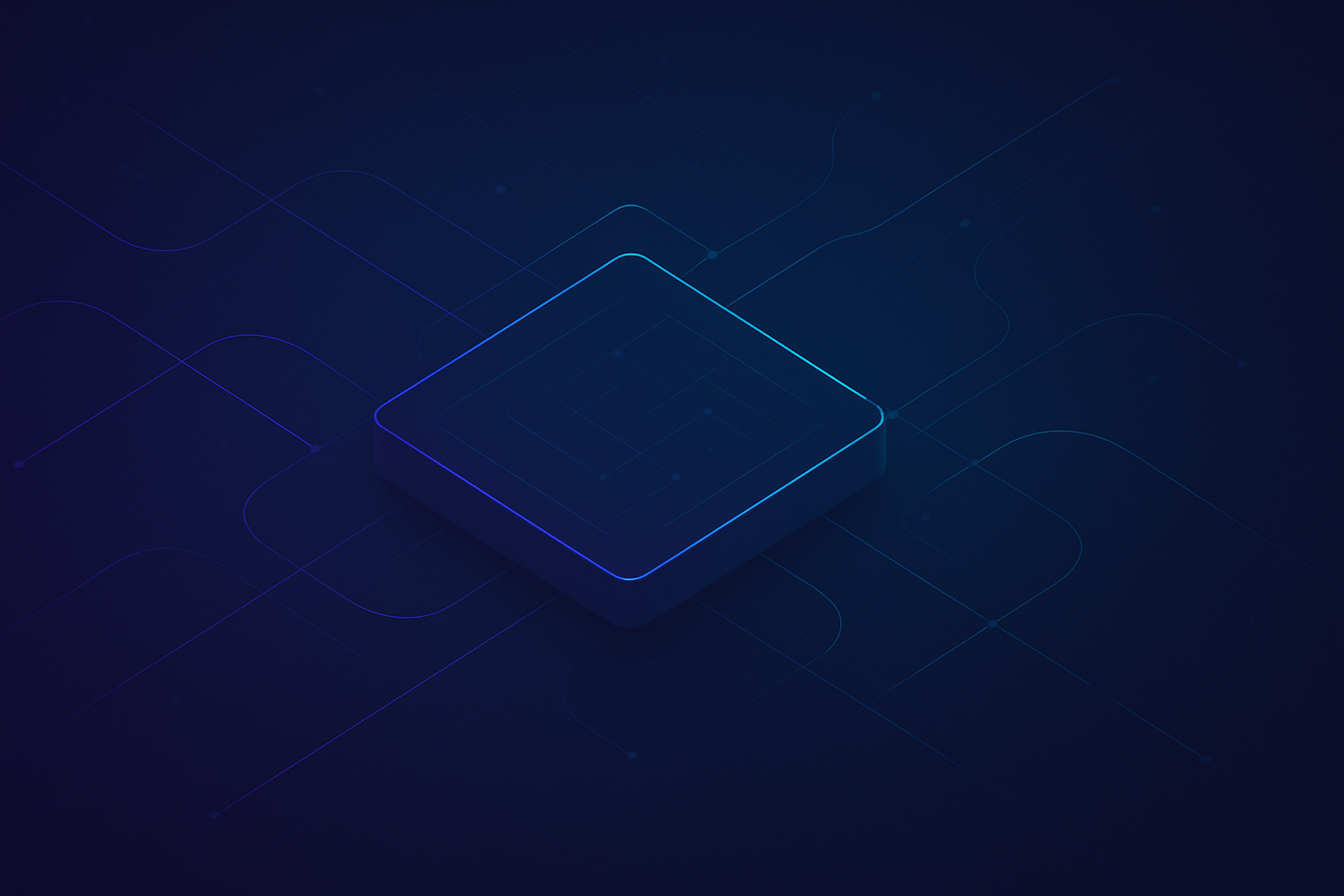
BeyondDataImporter for Microsoft Dynamics 365 Business Central provides an interface called "BYD DI IProcessor" which allows for streamlined and efficient data import into Business Central tables.
Wondering how fast an implementation could be?
Write an enumextension:
enumextension 60000 "PC PostCode" extends "BYD DI Processor"
{
value(60000; PostCode)
{
Caption = 'Post Code';
Implementation = "BYD DI IProcessor" = "PC Post Code Processor";
}
}
And implement the following interface:
interface "BYD DI IProcessor"
{
procedure GetTableNo(): Integer;
procedure GetTemplateFields(var Rec: Record "BYD DI Template Field" temporary);
procedure OnChunkLoaded(Rec: Record "BYD DI Template"; Chunk: JsonArray);
procedure OnImportFinished(Rec: Record "BYD DI Template");
}
Here’s a breakdown of the key components and functionalities:
1. Interface Implementation:
"BYD DI IProcessor"
indicates that this codeunit conforms to the interface, requiring specific methods to be implemented for processing data.
2. Procedures and Functions:
GetTableNo()
: Returns the ID of the"Post Code"
table.GetTemplateFields(...)
: Sets up the fields used in the import, including required/optional fields, and any relations.OnChunkLoaded(...)
: Called when a chunk of data is loaded.ProcessChunk(...)
: Processes each piece of the JSON data and maps it to the "Post Code" table.OnImportFinished(...)
: Finalizes the import and reports created/updated records.
3. Data Processing:
ProcessChunk
usesRecordRef
andFieldRef
to handle dynamic field assignment based on field type (Integer, Decimal, Boolean, Date, etc.).- Uses
ConfigValidateMgt.EvaluateTextToFieldRef
for type-safe conversion and validation.
4. Error Handling and Updates:
- Checks for existing records by key (e.g. "Code" + "City") and updates or inserts as needed.
- Tracks how many records were inserted/updated.
5. Performance Optimization:
- Uses temporary tables and indirect references for efficient batch handling.
- Ideal for large imports and background jobs.
And this is how it looks like:
Take our implementation code for free:
https://github.com/byndit/DataImporter-PostCode
Overall, the codeunit is designed for efficiency and flexibility, allowing easy adaptation for importing different datasets by merely implementing the necessary interface and adjusting field mappings and processing logic.